The third semester is about to end of my MBA and it's time to spend some time on the MBA research. Selecting a research topic is difficult. Doing literature review and developing on the thesis is even harder. After a chat which I had with Dr. Sanjiva Weerawarana I decided that I should select a topic which would be useful for WSO2, my organization that I work for as well and therefore thought of touching the area of marketing open-source software. I have no clue on where to start and at the moment am browsing through the web and collecting related magazines, articles which I think might be useful for me.
I wish my father was alive because I'm sure I would have had great support from him since he has done similar kinds of stuff and helped a lot of other people who have done their research projects. He did his PhD on radiochemistry and his research paper is available on the web but unfortunately I cannot access is since I have to pay in order to download it.
I could have got some help from my dear old sister who is a medical doctor at the University of Ragama but alas she too is doing her PhD these days and I don't think I will get any help from her either (She too has written some articles on her research).
So I guess I'm alone on this and will have to do it on my own. Well of course we are expected to do it on our own but it would have been great if I had someone who's close to me who would at least would understand my stress at a time like this. I know that there will be a lot of people at office to help me out but it won't be the same. We will see how it goes. My only hope is to be able to complete it by 2009 and graduate with the rest of the batch. :-)
Sunday, October 26, 2008
Friday, October 24, 2008
Creating your own Web Service with different session scopes
When creating a Web service you can create Web services of different session scopes. There are four session types in Axis2 such as,
1. SOAP Session Scope
2. Transport Session Scope
3. Request Scope
4. Application Session Scope
I will briefly explain how one can create Web service with each of these different session scopes.
Once you have created your Java class and complied it, you will need to write a services.xml before converting it into an archive file.
In order to create a service with a SOAP session scope all you have to do is add the following to your services.xml
scope="soapsession"
I will be providing a sample service.xml for your reference.
1. SOAP Session Scope
2. Transport Session Scope
3. Request Scope
4. Application Session Scope
I will briefly explain how one can create Web service with each of these different session scopes.
Once you have created your Java class and complied it, you will need to write a services.xml before converting it into an archive file.
In order to create a service with a SOAP session scope all you have to do is add the following to your services.xml
scope="soapsession"
E.g.:- <service name="<service_name>" scope="soapsession">
I will be providing a sample service.xml for your reference.
<service name="Adding" scope="soapsession">
<description>
This service is created to add two numbers.
</description>
<parameter name="ServiceClass" locked="false">com.math.add.Adding</parameter>
<operation name="add">
<messageReceiver class="org.apache.axis2.rpc.receivers.RPCMessageReceiver"/>
</operation>
</service>
Tuesday, October 21, 2008
Creating your own 'Mediator'
Recently I was asked by Ruwan to verify an issue reported by some customer. In order to test the issue, I had to write a new mediator. Since I'm not much familiar with writing these sort of stuff, I was struggling hard to get it working and in the end Ruwan helped me out to write a simple mediator and also taught me how to test it with WSO2 ESB. I will briefly describe how this can be done easily.
Pre-requisites - You need to download and install the latest version of WSO2 ESB on your local machine. (Assume that you have downloaded the WSO2 ESB instance to C:\wso2esb-1.7. I will be referring to this folder as ESB_HOME from this point onwards)
Step 1 - Create a mediator class
As the initial step I wrote a small java class which extends the method AbstractMediator as below.
Assume that you have your compiled java class at C:\ESB\mediator\testMediator\org\wso2\esb\client\TestMediator.class
Step 2 - Creating the JAR file
After compiling the class, I created a JAR file using the compiled class.
Open a new command prompt and go to the folder which you have the compiled package (E.g.:- C:\ESB\mediator\testMediator) and type the following command
Once you type this command it would create the JAR file testMediator.jar at the current location (E.g.:- C:\ESB\mediator\testMediator )
Step 3 - Creating the synapse configuration
In order to test the newly created mediator you can use a simple configuration as below. You can call the mediator which you created using a class mediator ( E.g.:- <class name="org.wso2.esb.client.TestMediator"/> ).
Step 4 - Starting the WSO2 ESB server with the created configuration
Copy this configuration to the ESB_HOME\webapp\WEB-INF\classes\conf and start the WSO2 ESB server. To do this, go to ESB_HOME\bin and run the command wso2-esb.bat
Step 5 - Creating the client to invoke the service
Assume that you have created a service (For you reference I will be attaching the service Adding1.aar which I have created) and deploy it in ESB_HOME\samples\axis2Server\repository\services. Go to the folder ESB_HOME\samples\axis2Server and start the SimpleAxis2Server by executing the executable bat file 'axis2server.bat'.
Now create a client as below and invoke the service deployed through WSO2 ESB.
Step 6 - Verifying through viewing the logs
Once you invoke the client, the logs displayed on the WSO2 ESB console will show how the class mediator has been invoked
As you can see only the Class mediator is invoked and non of the mediators specified after wards are not invoked. The reason for this is that when creating the mediator, you have set 'return' to false. Therefore when the Class mediator is invoked none of the mediators available after that Class mediator will not be invoked. If you need to invoke the other mediators that are available after the Class mediator, you have to set 'return' to true. Try this out by yourself. Once you execute the client, it should invoke the Class mediator first and the the Log mediator and after that the rest of the mediators specified.
Pre-requisites - You need to download and install the latest version of WSO2 ESB on your local machine. (Assume that you have downloaded the WSO2 ESB instance to C:\wso2esb-1.7. I will be referring to this folder as ESB_HOME from this point onwards)
Step 1 - Create a mediator class
As the initial step I wrote a small java class which extends the method AbstractMediator as below.
package org.wso2.esb.client;
import org.apache.axiom.soap.SOAPFault;
import org.apache.synapse.MessageContext;
import org.apache.synapse.mediators.AbstractMediator;
public class TestMediator extends AbstractMediator{
public boolean mediate(MessageContext synCtx) {
SOAPFault fault = synCtx.getEnvelope().getBody().getFault();
System.out.println(fault.getCode().getText());
System.out.println(fault.getReason().getText());
return false;
}
}
Assume that you have your compiled java class at C:\ESB\mediator\testMediator\org\wso2\esb\client\TestMediator.class
Step 2 - Creating the JAR file
After compiling the class, I created a JAR file using the compiled class.
Open a new command prompt and go to the folder which you have the compiled package (E.g.:- C:\ESB\mediator\testMediator) and type the following command
jar cf testMediator.jar org
Once you type this command it would create the JAR file testMediator.jar at the current location (E.g.:- C:\ESB\mediator\testMediator )
Step 3 - Creating the synapse configuration
In order to test the newly created mediator you can use a simple configuration as below. You can call the mediator which you created using a class mediator ( E.g.:- <class name="org.wso2.esb.client.TestMediator"/> ).
<definitions xmlns="http://ws.apache.org/ns/synapse">
<registry provider="org.wso2.esb.registry.ESBRegistry">
<parameter name="root">file:registry/</parameter>
<parameter name="cachableDuration">15000</parameter>
</registry>
<sequence name="main">
<sequence key="sampleSequence"/>
</sequence>
<sequence name="fault">
<log level="full">
<property name="MESSAGE" value="Executing default "fault" sequence"/>
<property name="ERROR_CODE" expression="get-property('ERROR_CODE')"/>
<property name="ERROR_MESSAGE" expression="get-property('ERROR_MESSAGE')"/>
</log>
<drop/>
</sequence>
<sequence name="sampleSequence" onError="soap11_fault">
<in>
<send>
<endpoint>
<address format="soap11" uri="http://localhost:9000/soap/Adding1"/>
</endpoint>
</send>
</in>
<out>
<class name="org.wso2.esb.client.TestMediator"/>
<log level="full"/>
<class name="org.wso2.esb.client.TestMediator"/>
<send/>
</out>
</sequence>
<sequence name="soap11_fault">
<log level="full" separator=","/>
<makefault version="soap11">
<code xmlns:sf11="http://schemas.xmlsoap.org/soap/encoding/" value="sf11:VersionMismatch"/>
<reason value="Exception occurred when transforming the request/response"/>
</makefault>
<log level="full"/>
<header name="To" action="remove"/>
<property name="RESPONSE" value="true"/>
</sequence>
</definitions>
Step 4 - Starting the WSO2 ESB server with the created configuration
Copy this configuration to the ESB_HOME\webapp\WEB-INF\classes\conf and start the WSO2 ESB server. To do this, go to ESB_HOME\bin and run the command wso2-esb.bat
Step 5 - Creating the client to invoke the service
Assume that you have created a service (For you reference I will be attaching the service Adding1.aar which I have created) and deploy it in ESB_HOME\samples\axis2Server\repository\services. Go to the folder ESB_HOME\samples\axis2Server and start the SimpleAxis2Server by executing the executable bat file 'axis2server.bat'.
Now create a client as below and invoke the service deployed through WSO2 ESB.
package org.wso2.esb.client;
import org.apache.axiom.om.OMAbstractFactory;
import org.apache.axiom.om.OMElement;
import org.apache.axiom.om.OMFactory;
import org.apache.axiom.om.OMNamespace;
import org.apache.axis2.AxisFault;
import org.apache.axis2.addressing.EndpointReference;
import org.apache.axis2.client.Options;
import org.apache.axis2.client.ServiceClient;
public class AxiomClient {
public static void main(String[] args)throws AxisFault{
Options options = new Options();
options.setTo(new EndpointReference("http://localhost:8280/soap"));
options.setAction("urn:add");
ServiceClient sender = new ServiceClient();
sender.setOptions(options);
OMElement result = sender.sendReceive(getPayload());
System.out.println(result);
}
private static OMElement getPayload() {
OMFactory fac = OMAbstractFactory.getOMFactory();
OMNamespace omNs = fac.createOMNamespace("http://service.esb.wso2.org", "ns");
OMElement method = fac.createOMElement("add", omNs);
OMElement value1 = fac.createOMElement("x", omNs);
OMElement value2 = fac.createOMElement("y", omNs);
value1.addChild(fac.createOMText(value1, "10"));
value2.addChild(fac.createOMText(value2, "10"));
method.addChild(value1);
method.addChild(value2);
return method;
}
}
Step 6 - Verifying through viewing the logs
Once you invoke the client, the logs displayed on the WSO2 ESB console will show how the class mediator has been invoked
[HttpClientWorker-1] DEBUG InMediator Start : In mediator
[HttpClientWorker-1] DEBUG InMediator Current message is a response - skipping child mediators
[HttpClientWorker-1] DEBUG InMediator End : In mediator
[HttpClientWorker-1] DEBUG OutMediator Start : Out mediator
[HttpClientWorker-1] DEBUG OutMediator Current message is outgoing - executing child mediators
[HttpClientWorker-1] DEBUG OutMediator Sequence <OutMediator> :: mediate()
[HttpClientWorker-1] DEBUG ClassMediator Start : Class mediator
[HttpClientWorker-1] DEBUG ClassMediator invoking : class org.wso2.esb.client.TestMediator.mediate()
axis2ns8:Client
The service cannot be found for the endpoint reference (EPR) http://localhost:9000/soap/Adding1
[HttpClientWorker-1] DEBUG ClassMediator End : Class mediator
[HttpClientWorker-1] DEBUG OutMediator End : Out mediator
As you can see only the Class mediator is invoked and non of the mediators specified after wards are not invoked. The reason for this is that when creating the mediator, you have set 'return' to false. Therefore when the Class mediator is invoked none of the mediators available after that Class mediator will not be invoked. If you need to invoke the other mediators that are available after the Class mediator, you have to set 'return' to true. Try this out by yourself. Once you execute the client, it should invoke the Class mediator first and the the Log mediator and after that the rest of the mediators specified.
Tuesday, October 7, 2008
Deploying WSO2 ESB on an IBM WebSphere Application Server
Applies To
1. WSO2 Enterprise Service Bus (ESB) Version 1.7
2. WebSphere Application Server Version 6.1
Download the WebSphere Application Server Version 6.1 and execute the setup to install it on your local machine.
Before deploying the WSO2 ESB application we need to create a profile through the Profile Management Tool. This is described in detail in the tutorial Running WSO2 WSAS on an IBM WebSphere Application Server under the heading Creating an IBM WebSphere Application Server Profile by Upul Godage.
NOTE: Make sure you set the HTTPS transport port to 9444.
Once you have created the profile start the application server through Start -> All Programs -> IBM WebSphere -> Application Server V6.1 -> Profiles -> [your_profile] -> Start the server.
When the server is started successfully access the Administrative Console through Start -> All Programs -> IBM WebSphere -> Application Server V6.1 -> Profiles -> [your_profile] -> Administrative Console.
Once clicked it would open up a web browser. Enter the username and password that we gave in the Administrative Security step when creating the profile and then click on the button Log in.
Download WSO2 ESB version 1.7 from the WSO2 site and extract it to a preferred location of yours. (E.g.:- C:\wso2esb-1.7.1). From this point onwards I will be referring to this folder as ESB_HOME. Before creating the war file you need to change some of the configuration files. Let us look at these files one by one.
1. axis2.xml
a) Open the axis2.xml located at ESB_HOME/webapp/WEB-INF/classes/conf
b) Search all the places where it specifies the jks file locations and replace them with the absolute paths.
2. web.xml
a) Open the web.xml file located at ESB_HOME/webapp/WEB-INF
b) Specify the absolute location of the ESB_HOME
Next we have to start up a Derby server for WSO2 ESB to connect to. Since we have an issue in getting the Derby server which resides in Websphere up and running, we will be starting an external Derby server.
To do this, download the latest Derby binary and extract to a preferred location of yours. For testing purposes we will be using version 10.3.1.4. Then go to the folder DERBY_HOME/bin folder and type the following command.
To direct the WSO2 ESB to connect to the external Derby server you need to set the StartEmbeddedDerby property to 'false' of the ESB_HOME/webapp/WEB-INF/classes/conf/server.xml file
You are all set to create the war file. Now go to the folder 'webapp' of your ESB_HOME and open a command window.
Type the following command and it will create esb.war file in ESB_HOME/webapp folder
Follow the step described below to successfully install the WSO2 ESB application.
1. Once logged in to the Administrative Console, click on Applications -> Install New Application
2. Under Preparing for the application installation browse for the esb.war file that you created above. Specify the context root as esb. If you need to see the steps when installing the WSO2 ESB Application, select the combo box option Show me all installation options and parameters and click Next.
3. From the page loaded to generate default bindings and mappings click on Next without changing any options
4. Click on Continue given under Application Security Warnings page
5. When Install New Application -> Select installation options is loaded specify a name that you prefer as the Application name. Tick the check box Enable class reloading and specify the Reload interval in seconds as 60 seconds and then click on Next
6. Under Install New Application -> Map modules to servers do not select any options and just click on the button Next
7. When the page Install New Application -> Provide JSP reloading options for Web modules loads click on the button Next without selecting anything
8. Next do not select any options given under the page Install New Application -> Map shared libraries and simply click on Next
9. When the page Install New Application -> Initialize parameters for servlets is loaded check whether the specified parameters are correct and click on Next
10. Click on Next without selecting any options when Install New Application -> Map virtual hosts for Web modules page is loaded
11. Verify whether the value given as the context root is correct in the page Install New Application -> Map context roots for Web modules and click on Next
12. When the Install New Application -> Summary page loads, go through the values which you have specified and if they are correct click on Finish
Once you click on Continue you will get a message as follows. Therefore click on Save and the application will be installed successfully.
Go to Applications -> Enterprise Applications and click on the esb entry from the list of applications available.
Then refer the section Configuring IBM WebSphere Application Server in the tutorial Running WSO2 WSAS on an IBM WebSphere Application Server by Upul Godage.
Follow the steps specified and once you are done with setting all the properties select the esb application from the Enterprise Applications list and click on Start. This will start the WSO2 ESB server. If the server starts successfully you can try accessing the WSO2 ESB Administration Console through https://localhost:9444/esb
1. WSO2 Enterprise Service Bus (ESB) Version 1.7
2. WebSphere Application Server Version 6.1
Downloading and Installing WebSphere Application Server
Download the WebSphere Application Server Version 6.1 and execute the setup to install it on your local machine.
Creating a profile
Before deploying the WSO2 ESB application we need to create a profile through the Profile Management Tool. This is described in detail in the tutorial Running WSO2 WSAS on an IBM WebSphere Application Server under the heading Creating an IBM WebSphere Application Server Profile by Upul Godage.
NOTE: Make sure you set the HTTPS transport port to 9444.
Starting the Websphere Application server
Once you have created the profile start the application server through Start -> All Programs -> IBM WebSphere -> Application Server V6.1 -> Profiles -> [your_profile] -> Start the server.
Login into the Administrative Console
When the server is started successfully access the Administrative Console through Start -> All Programs -> IBM WebSphere -> Application Server V6.1 -> Profiles -> [your_profile] -> Administrative Console.
Once clicked it would open up a web browser. Enter the username and password that we gave in the Administrative Security step when creating the profile and then click on the button Log in.
E.g.:-
User ID: admin
Password: admin
Downloading WSO2 ESB and creating a war file
Download WSO2 ESB version 1.7 from the WSO2 site and extract it to a preferred location of yours. (E.g.:- C:\wso2esb-1.7.1). From this point onwards I will be referring to this folder as ESB_HOME. Before creating the war file you need to change some of the configuration files. Let us look at these files one by one.
1. axis2.xml
a) Open the axis2.xml located at ESB_HOME/webapp/WEB-INF/classes/conf
b) Search all the places where it specifies the jks file locations and replace them with the absolute paths.
E.g.:-
Change
<Location>webapp/WEB-INF/classes/conf/identity.jks</Location>
to
<Location>C:/wso2esb-1.7.1/webapp/WEB-INF/classes/conf/identity.jks</Location>
2. web.xml
a) Open the web.xml file located at ESB_HOME/webapp/WEB-INF
b) Specify the absolute location of the ESB_HOME
E.g.:-
Replace
<init-param>
<param-name>esb.home</param-name>
<param-value>.</param-value>
</init-param>
with
<init-param>
<param-name>esb.home</param-name>
<param-value>C:/wso2esb-1.7.1</param-value>
</init-param>
Next we have to start up a Derby server for WSO2 ESB to connect to. Since we have an issue in getting the Derby server which resides in Websphere up and running, we will be starting an external Derby server.
To do this, download the latest Derby binary and extract to a preferred location of yours. For testing purposes we will be using version 10.3.1.4. Then go to the folder DERBY_HOME/bin folder and type the following command.
NetworkServerControl start -p 1528
To direct the WSO2 ESB to connect to the external Derby server you need to set the StartEmbeddedDerby property to 'false' of the ESB_HOME/webapp/WEB-INF/classes/conf/server.xml file
<StartEmbeddedDerby>false</StartEmbeddedDerby>
You are all set to create the war file. Now go to the folder 'webapp' of your ESB_HOME and open a command window.
Type the following command and it will create esb.war file in ESB_HOME/webapp folder
jar -cvf esb.war *
Installing the WSO2 ESB appliacation on IBM Websphere
Follow the step described below to successfully install the WSO2 ESB application.
1. Once logged in to the Administrative Console, click on Applications -> Install New Application
2. Under Preparing for the application installation browse for the esb.war file that you created above. Specify the context root as esb. If you need to see the steps when installing the WSO2 ESB Application, select the combo box option Show me all installation options and parameters and click Next.
3. From the page loaded to generate default bindings and mappings click on Next without changing any options
4. Click on Continue given under Application Security Warnings page
5. When Install New Application -> Select installation options is loaded specify a name that you prefer as the Application name. Tick the check box Enable class reloading and specify the Reload interval in seconds as 60 seconds and then click on Next
6. Under Install New Application -> Map modules to servers do not select any options and just click on the button Next
7. When the page Install New Application -> Provide JSP reloading options for Web modules loads click on the button Next without selecting anything
8. Next do not select any options given under the page Install New Application -> Map shared libraries and simply click on Next
9. When the page Install New Application -> Initialize parameters for servlets is loaded check whether the specified parameters are correct and click on Next
10. Click on Next without selecting any options when Install New Application -> Map virtual hosts for Web modules page is loaded
11. Verify whether the value given as the context root is correct in the page Install New Application -> Map context roots for Web modules and click on Next
12. When the Install New Application -> Summary page loads, go through the values which you have specified and if they are correct click on Finish
Once you click on Continue you will get a message as follows. Therefore click on Save and the application will be installed successfully.
Configuring the WSO2 ESB Application
Go to Applications -> Enterprise Applications and click on the esb entry from the list of applications available.
Then refer the section Configuring IBM WebSphere Application Server in the tutorial Running WSO2 WSAS on an IBM WebSphere Application Server by Upul Godage.
Follow the steps specified and once you are done with setting all the properties select the esb application from the Enterprise Applications list and click on Start. This will start the WSO2 ESB server. If the server starts successfully you can try accessing the WSO2 ESB Administration Console through https://localhost:9444/esb
Monday, October 6, 2008
Starting the Derby Network Server on a new port
I was testing WSO2 ESB on IBM Websphere and I could not get the Derby server inside Websphere running and therefore wanted to start a new Derby server outside Websphere. I was struggling to get this working till I came across a link which was specified by one of my colleges in one of the WSO2 ESB forum posts. In that article it clearly specifies how you can start a new Derby server on a new port.
NetworkServerControl start -p 1528
Generally when you start up WSO2 ESB it would start a Derby database which is embedded inside WSO2 ESB. Therefore if you want the ESB instance to listen to the new Derby server which you started on port 1528 then all you have to do is set the following property to false of the ESB_HOME/webapp/WEB-INF/classes/conf/server.xml file.
<StartEmbeddedDerby>false</StartEmbeddedDerby>
NetworkServerControl start -p 1528
Generally when you start up WSO2 ESB it would start a Derby database which is embedded inside WSO2 ESB. Therefore if you want the ESB instance to listen to the new Derby server which you started on port 1528 then all you have to do is set the following property to false of the ESB_HOME/webapp/WEB-INF/classes/conf/server.xml file.
<StartEmbeddedDerby>false</StartEmbeddedDerby>
Friday, October 3, 2008
How to disable chunking/keep-alive when using Synapse
How do you disable chunking/keep-alive? Many users have asked this question through the Synapse/WSO2 ESB mailing lists several times. This is possible through the Property mediator.
Generally chunking is enabled by default. If you need to disable chunking/keep-alive all you have to do is set the property "FORCE_HTTP_1.0" to "TRUE" in the scope axis2 using Property Mediator
<property name="FORCE_HTTP_1.0" value="true"/>
Generally chunking is enabled by default. If you need to disable chunking/keep-alive all you have to do is set the property "FORCE_HTTP_1.0" to "TRUE" in the scope axis2 using Property Mediator
Thursday, October 2, 2008
How to enable JMX monitoring support on Synapse
How do you enable JMX monitoring support on Synapse? This is a question that may arise in someone who would try out Synapse. In order to do this you will have to add the following line to the synapse.sh file
-Dcom.sun.management.jmxremote
Once this line is added you just need to start the Synapse server. When the Synapse server is started, open a new terminal window and type the command 'jconsole' and then you will be given the Monitoring & Management Console along with the available servers to connect
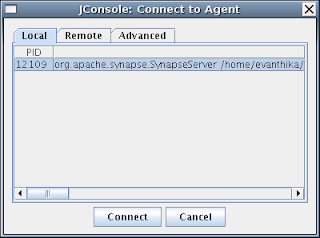
-Dcom.sun.management.jmxremote
Once this line is added you just need to start the Synapse server. When the Synapse server is started, open a new terminal window and type the command 'jconsole' and then you will be given the Monitoring & Management Console along with the available servers to connect
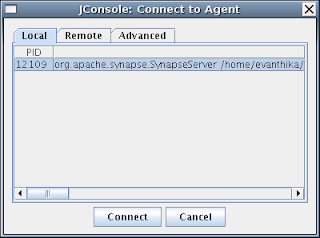
Subscribe to:
Posts (Atom)